Mastering Advanced C++
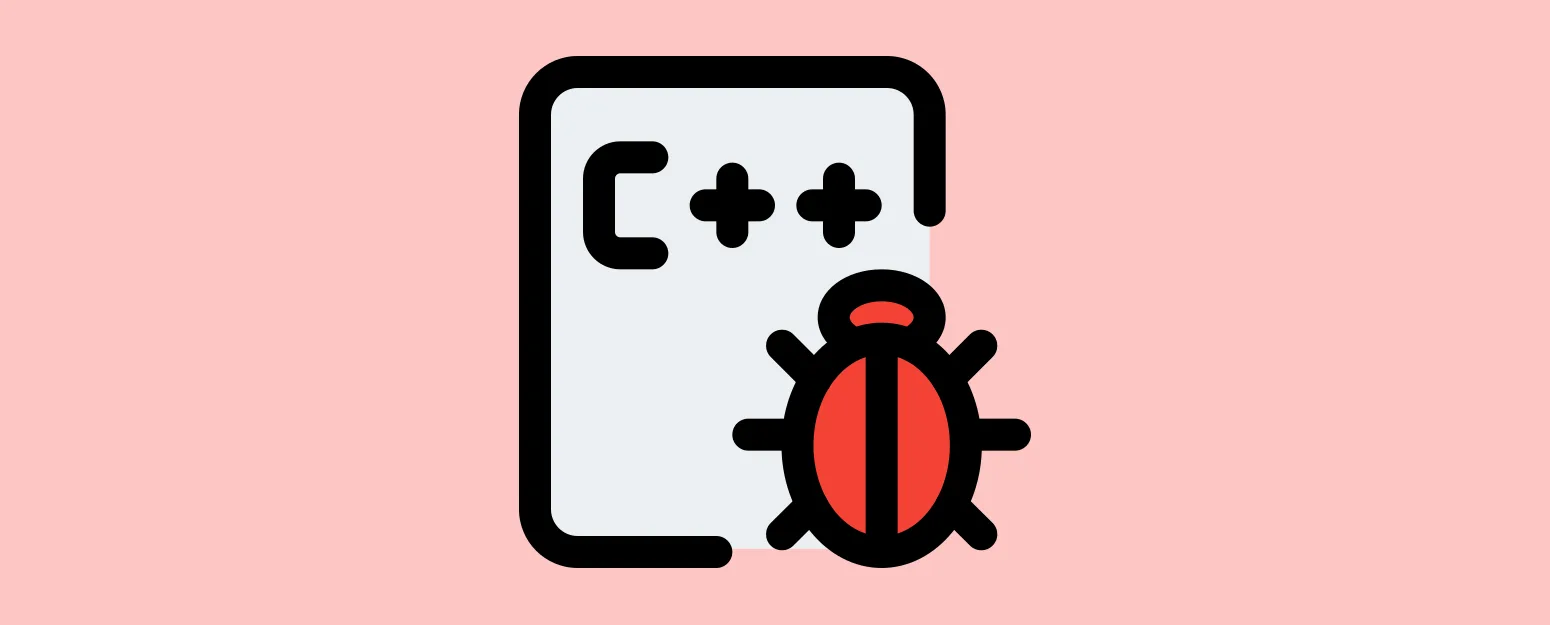
Embark on a transformative journey into the realm of Advanced C++. Elevate your programming prowess as you explore intricate concepts, powerful techniques, and cutting-edge features. Uncover the secrets of optimized performance, robust design patterns, and efficient data structures. With real-world examples and expert guidance, this learning experience will equip you to craft high-quality, innovative software solutions that stand out in the competitive landscape of modern development. Dive into Advanced C++ and unlock the potential to create code that not only excels in functionality but also dazzles with its elegance and ingenuity.
Basics of C++ Programming
Object-Oriented Programming (OOP) Principles
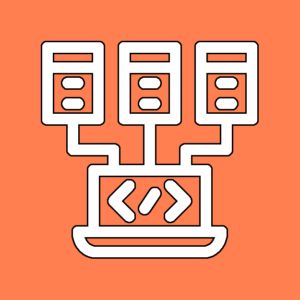
Objects
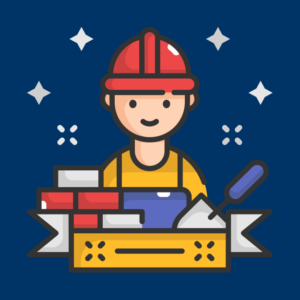
Destructors
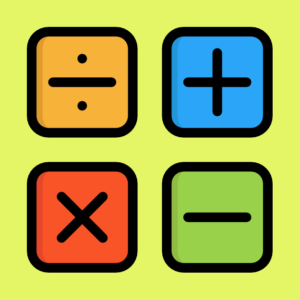
Overloading
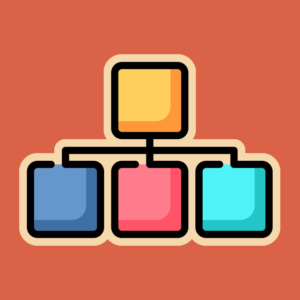
.
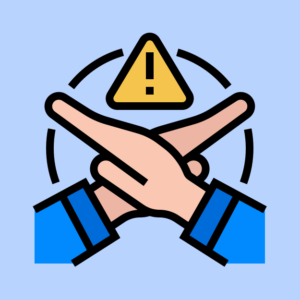
.
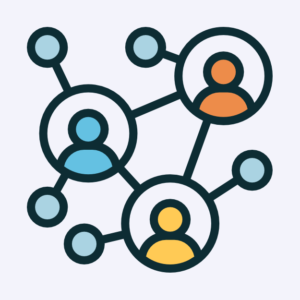
.
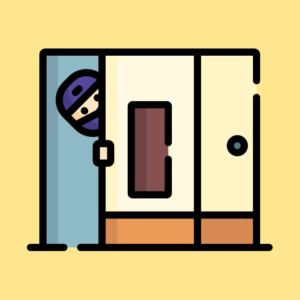
.
Standard Template Library (STL)
Memory Management
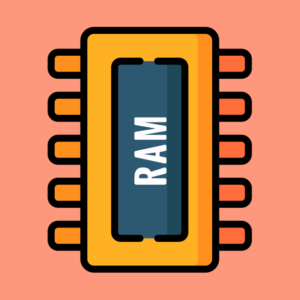
Memory
Allocation
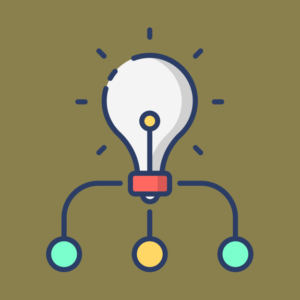
(Resource Acquisition
Is Initialization)
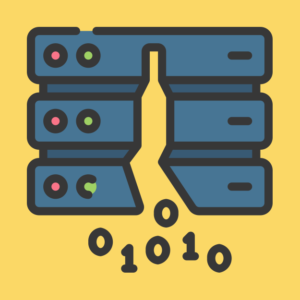
.
.
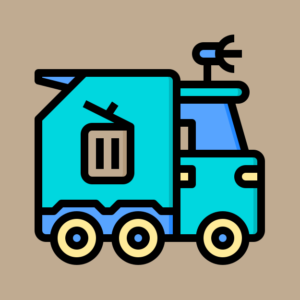
.
.
Exception Handling
File Handling
Standard C++ Library
Multithreading and Concurrency
C++11 Features
Best Practices and Design Patterns
C++ Compiler and Build Tools
Debugging and Profiling
C++ Frameworks and Libraries
Networking and Socket Programming
Graphics and Game Development
Embedded Systems and C++
C++ Best Practices for Performance
New Features and Enhancements
In conclusion, this comprehensive journey through various facets of C++ has provided you with a solid foundation and a deep understanding of this versatile programming language. From mastering its fundamentals to delving into advanced topics, you’ve acquired the knowledge and skills needed to excel in C++ development.
As you continue your coding odyssey, remember that learning is an ongoing process. The world of technology is ever-evolving, and staying up-to-date with the latest trends, tools, and features will be essential. Whether you’re crafting efficient algorithms, designing elegant user interfaces, or optimizing performance, your C++ expertise will empower you to create remarkable software solutions.
So, keep exploring, keep experimenting, and keep pushing the boundaries of what you can achieve with C++. With dedication and a thirst for knowledge, there’s no limit to the extraordinary things you can create. Whether you’re building applications, games, or complex systems, your journey through the world of C++ is just the beginning of an exciting and fulfilling adventure.